I got the titled error when setting up a new SharePoint 2010 farm and try to start up the User Profile Synchronization Service in Manage Services on Server. In this article I will describe my understanding to UPS and FIM service how do I troubleshoot it.
Here is the pattern for my problem. After I press on start “User Profile Synchronization Service" within “Manage Services on Server". It shows “starting" for about 10-15 minutes and then it change back to “Stopped" status.
The “User Profile Synchronization Service" is actually calling 2 windows services. You will find them by Windows Services console (key in services.msc to call this console):
Forefront Identity Manager Service (FIMService)
Forefront Identity Manager Synchronization Service (FIMSynchronizationService)
During the “User Profile Synchronization Service" is “starting", FIMSynchronizationService is changed from disabled to started. I see FIMService is trying to start (from disabled to starting) and then fail after a minutes.
At the same moment, I check the ULS log and filter with keyword “ILM" and see following:
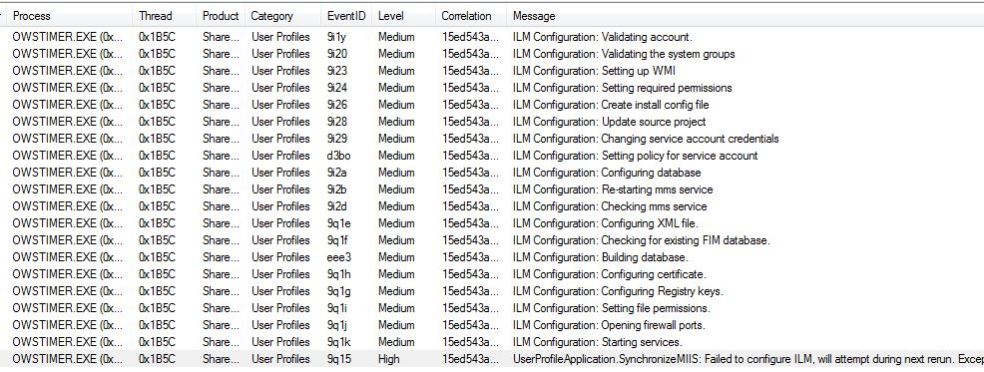
Above log have shown what FIMSynchronizationService is doing. In the log “Configuring database" I believe it is working with User Profile Sync DB as I can see the DB is modified the same time. It proved that FIMSynchronizationService have sufficient permission to the database.
In the last line, the full error message is “UserProfileApplication.SynchronizeMIIS: Failed to configure ILM, will attempt during next rerun. Exception: System.FormatException: Index (zero based) must be greater than or equal to zero and less than the size of the argument list."
The same pattern of logs will show up several times (sorry I am too lazy to count) and then FIMSynchronizationService will give up. After it gave up, “User Profile Synchronization Service" within “Manage Services on Server" will go back to “Stopped" status.
After hours of troubleshooting, I found this great article Best Practices for CRL Checking on SharePoint Servers. The blogger introduce how to handle CRL checking by SharePoint. If your SharePoint server is like mine, which DO NOT HAVE INTERNET ACCESS, I highly recommend you read it. Because Microsoft always assume your farm have Internet access and do not have official KB to tell you how to handle if you don’t.
Regarding this FIM problem, I solved by following steps:
- Launch gpedit.msc as admin on the SharePoint server
- Look for Local Computer policy > Windows Settings > Security settings > Public Key Policies
- Open Certificate Path Validation Settings, in the Network retrieval tab check “Define the policy settings" and uncheck the option to “Automatically update certificates in the Microsoft Root Certificate Program (recommended)."
- Run gpupdate /force
I am sure User Profile Synchronization Service is a permanent headache to all SharePoint administrators. If you search on Internet you will get tons of related error message and various solution. The same UPS will still in use for SharePoint 2013. I heard SharePoint 2016 will replace it with new function but I don’t have chance to play on new toy yet.